Technical Document - Function Description - BLE(Bluetooth Low Enegy)
Table of Contents
1. Overview
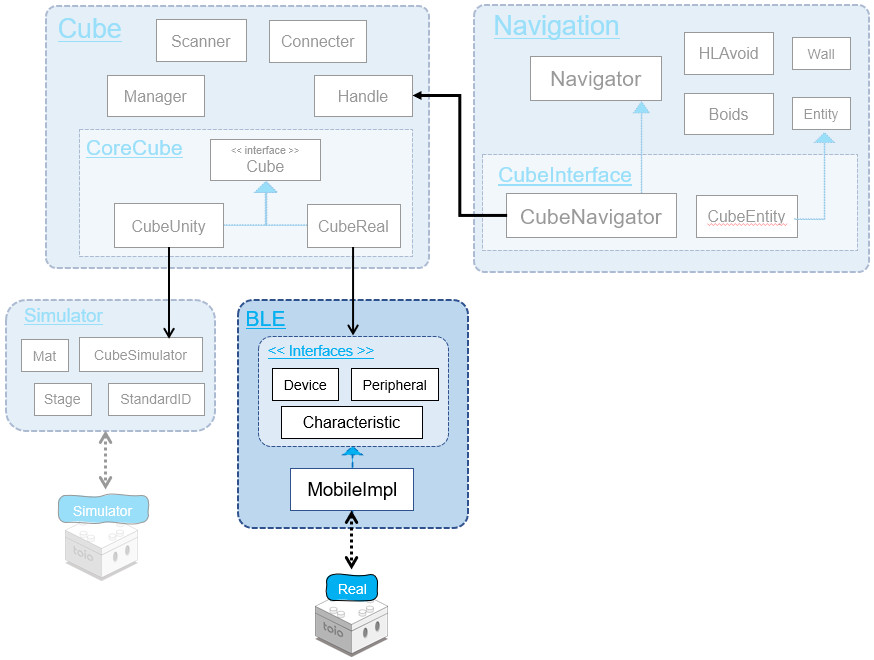
BLE module group is a group of modules that provide Bluetooth Low Enegy (BLE) communication functions in an abstract manner via an interface. By implementing all BLE classes through the interface, toio™ programming can be done independently of the communication architecture. Therefore, it is possible to replace them with communication modules other than BLE by developing new inheritance classes.
The directory structure is as shown in the figure below.
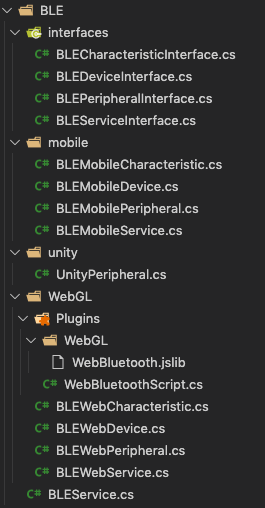
BLE +---------------------------------+ BLE root directory
├── interfaces +----------------------+ interface directory
│ ├── BLECharacteristicInterface.cs + Characteristic (functional unit) interface
│ ├── BLEDeviceInterface.cs +-------+ Interface that provides BLE functionality for terminals
│ ├── BLEPeripheralInterface.cs +---+ Peripheral (connection terminal) interface
│ └── BLEServiceInterface.cs +------+ Interface as the first point of contact for BLE processing
├── mobile +--------------------------+ Mobile Implementation Directory
│ ├── BLEMobileCharacteristic.cs +--+ Characteristic (functional unit) mobile implementation class
│ ├── BLEMobileDevice.cs +----------+ Class that provides BLE functionality for mobile devices
│ ├── BLEMobilePeripheral.cs +------+ Peripheral (connected terminal) mobile implementation class
│ └── BLEMobileService.cs +---------+ Mobile implementation class that is the first point of contact for BLE processing
├── unity +---------------------------+ Unity Implementation Directory
│ └── UnityPeripheral.cs +----------+ Peripheral(GameObject)Unity implementation class
├── WebGL +---------------------------+ WebGL Implementation Directory
│ ├── Plugins +---------------------+ Plug-in directory for WebGL
│ │ ├── WebGL +-------------------+ Plug-in directory for WebGL
│ │ │ └── WebBluetooth.jslib +--+ Script that interacts with WebGLTemplates/webble/webble.js
│ │ └── WebBluetoothScript.cs +---+ Class that provides plug-in functionality (interacts with WebBluetooth.jslib)
│ ├── BLEWebCharacteristic.cs +-----+ Characteristic (functional unit) WebGL implementation class
│ ├── BLEWebDevice.cs +-------------+ Class that provides BLE functionality for WebGL
│ ├── BLEWebPeripheral.cs +---------+ Peripheral (connected terminal) WebGL implementation class
│ ├── BLEWebService.cs +------------+ WebGL implementation class that serves as the first window for BLE processing
└── BLEService.cs +-------------------+ Singleton class, the first point of contact for the entire BLE
2. Structure of BLE class
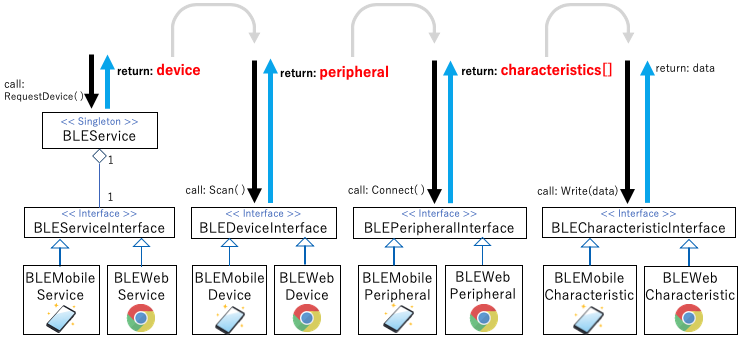
BLEService
Singleton class as the first window for BLE functionality
BLEService.Instance.SetImplement()Set the internal implementation class by putting the implementation instance in the function.
Implementation code
public class BLEService : GenericSingleton<BLEService>
{
public bool hasImplement { get; private set; }
public BLEService()
{
this.hasImplement = false;
}
private BLEServiceInterface impl;
public void SetImplement(BLEServiceInterface impl)
{
this.impl = impl;
this.hasImplement = true;
}
public void RequestDevice(Action<BLEDeviceInterface> action)
{
this.impl.RequestDevice(action);
}
public async UniTask Enable(bool enable, Action action)
{
await this.impl.Enable(enable, action);
}
public void DisconnectAll()
{
this.impl.DisconnectAll();
}
}
BLEServiceInterface
Interface that serves as the first window for BLE processing
It provides a function to acquire the Device interface that provides BLE function of the operating terminal.
Interface code
public interface BLEServiceInterface
{
void RequestDevice(Action<BLEDeviceInterface> action);
void DisconnectAll();
UniTask Enable(bool enable, Action action);
}
BLEDeviceInterface
BLE function interface of the operating terminal
Its main role is to access BLE functions and perform scanning operations.
Interface code
public interface BLEDeviceInterface
{
void Scan(String[] serviceUUIDs, bool rssiOnly, Action<BLEPeripheralInterface> action);
void StopScan();
UniTask Disconnect(Action action);
UniTask Enable(bool enable, Action action);
}
BLEPeripheralInterface
Interface for scanned BLE devices
Its main role is to perform the connection process to the scanned BLE device.
Interface code
public interface BLEPeripheralInterface
{
string[] serviceUUIDs { get; }
string device_address { get; }
string device_name { get; }
float rssi { get; }
bool isConnected { get; }
void Connect(Action<BLECharacteristicInterface> characteristicAction);
void AddConnectionListener(string key, Action<BLEPeripheralInterface> action);
void RemoveConnectionListener(string key);
void ConnectionNotify(BLEPeripheralInterface peri);
}
BLECharacteristicInterface
Interface for each function of the scanned BLE device
The main role is to perform write/read operations for each function of BLE device.
Interface code
public interface BLECharacteristicInterface
{
string deviceAddress { get; }
string serviceUUID { get; }
string characteristicUUID { get; }
void ReadValue(Action<string, byte[]> action);
void WriteValue(byte[] data, bool withResponse);
void StartNotifications(Action<byte[]> action);
void StopNotifications();
}
3. How Communication Works
3.1. Search and connect
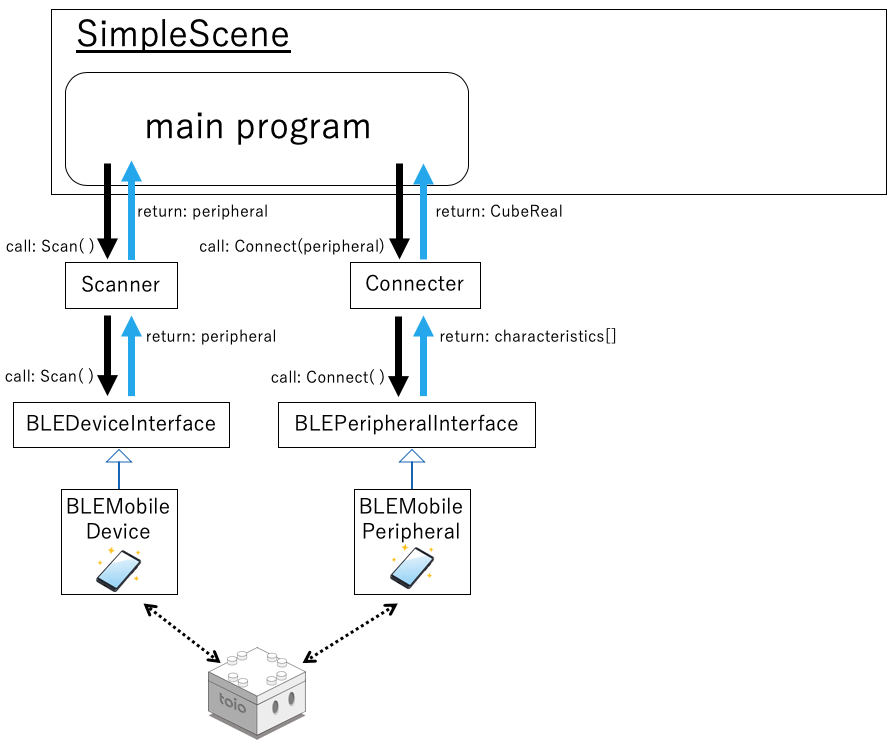
Search
For an overview of the Scanner class, refer to here.
This section describes BLE processing of the Scanner class.
The scan roughly follows the steps below.
- Call the RequestDevice function to obtain the BLDeviceInterface variable.
- Start scanning for CoreCube Identification ID.
- End the scan when the conditions (number of connections, waiting time) are met
- Return BLEPeripheralInterface variable obtained by scanning
Connect
The Connecter class is used for the connection. Please refer to the cube documentation, which describes most of the details.
The following points should be added to BLE process.
- Immediately after Cube variable is created, it calls Subscribe to automatic notifications (cube.StartNotifications) to start subscribing to automatic notifications such as coordinates and buttons.
3.2. Send and Receive
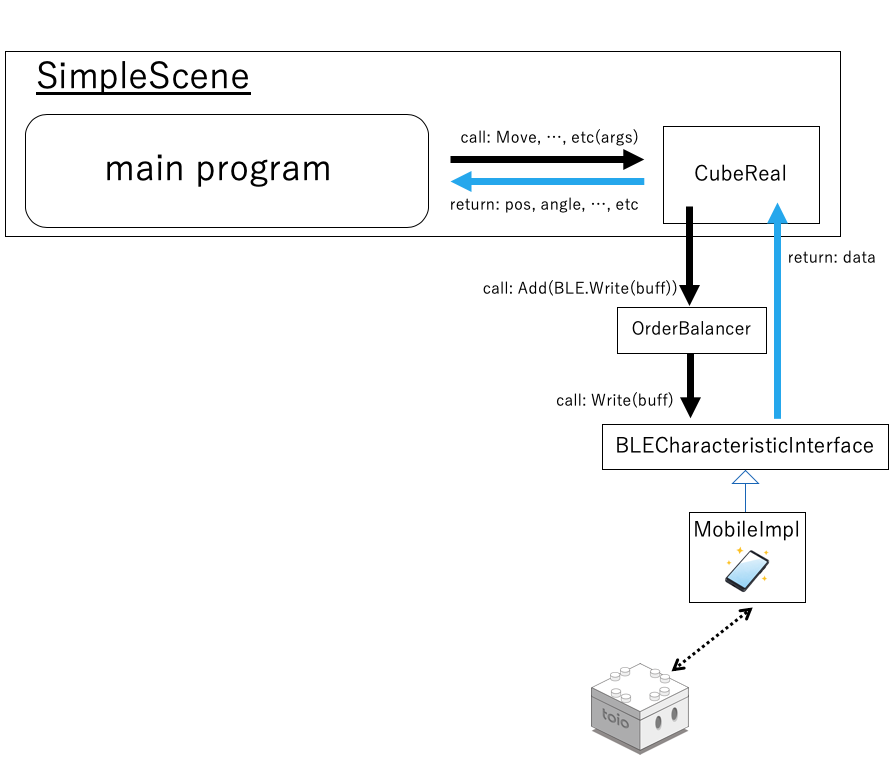
Send
The sending call itself is made from a derived class of CubeReal, see cube documentation.
Receive
The subscribed information will be automatically notified by Subscribe to automatic notifications (cube.StartNotifications) done immediately after the connection process.